What is ASP.Net?
ASP.Net is a web development platform, which provides a programming model, a comprehensive software infrastructure and various services required to build up robust web application for PC, as well as mobile devices.
ASP.Net is a part of Microsoft .Net platform. ASP.Net applications could be written in either of the following languages:
- C#
- Visual Basic .Net
- Jscript
- J#
ASP.Net framework helps in storing the information regarding the state of the application, which consists of:
- Page state
- Session state
The page state is the state of the client, i.e., the content of various input fields in the web form. The session state is the collective obtained from various pages the user visited and worked with, i.e., the overall session state.
ASP.Net Environment Setup:
The key development tool for building ASP.Net applications and front ends is Visual Studio.
Visual Studio is an integrated development environment for writing, compiling and debugging the code. It provides a complete set of development tools for building ASP.Net web applications, web services, desktop applications and mobile applications.
When you start a new web site, ASP.NET provides the starting folders and files for the site, including two files for the first web form of the site.
The file named Default.aspx contains the HTML and asp code that defines the form, and the file named Default.aspx.cs (for C# coding) or the file named Default.aspx.vb (for vb coding) contains the code in the language you have chosen and this code is responsible for the form's works.
A typical ASP.Net application consists of many items: the web content files (.aspx), source files (e.g., the .cs files), assemblies (e.g., the .dll files and .exe files), data source files (e.g., .mdb files), references, icons, user controls and miscellaneous other files and folders. All these files that make up the website are contained in a Solution.
Typically a project contains the following content files:
- Page file (.aspx)
- User control (.ascx)
- Web service (.asmx)
- Master page (.master)
- Site map (.sitemap)
- Website configuration file (.config)
The application is run by selecting either Start or Start Without Debugging from the Debug menu, or by pressing F5 or Ctrl-F5. The program is built i.e. the .exe or the .dll files are generated by selecting a command from the Build menu.
ASP.Net Life Cycle:
ASP.Net life cycle specifies, how:
- ASP.Net processes pages to produce dynamic output
- The application and its pages are instantiated and processed
- ASP.Net compiles the pages dynamically
The ASP.Net life cycle could be divided into two groups:
- Application Life Cycle: When user makes a request for accessing application resource, a page. Browser sends this request to the web server and it request passes through several stages before server response gets back.
- Page Life Cycle: When a page is requested, it is loaded into the server memory, processed and sent to the browser. Then it is unloaded from the memory. At each of this steps, methods and events are available, which could be overridden according to the need of the application. In other words, you can write your own code to override the default code.
ASP.Net Example:
An ASP.Net page is also a server side file saved with the .aspx extension. It is modular in nature and can be divided into the following core sections:
- Page directives
- Code Section
- Page Layout
Page directives:
The page directives set up the environments for the page to run. The @Page directive defines page-specific attributes used by the ASP.Net page parser and compiler. Page directives specify how the page should be processed, and which assumptions are to be taken about the page.
It allows importing namespaces, loading assemblies and registering new controls with custom tag names and namespace prefixes. We will discuss all of these concepts in due time.
Code Section:
The code section provides the handlers for the page and control events along with other functions required. We mentioned that, ASP.Net follows an object model. Now, these objects raises events when something happens on the user interface, like a user clicks a button or moves the cursor. How these events should be handled? That code is provided in the event handlers of the controls, which are nothing but functions bound to the controls.
The code section or the code behind file provides all these event handler routines, and other functions used by the developer. The page code could be precompiled and deployed in the form of a binary assembly.
Page Layout:
The page layout provides the interface of the page. It contains the server controls, text, inline JavaScript and HTML tags:
The following code snippet provides a sample ASP.Net page explaining pafe directives, code section and page layout written in C#:
<!-- directives --> <% @Page Language="C#" %> <!-- code section --> <script runat="server"> private void convertoupper(object sender, EventArgs e) { string str = mytext.Value; changed_text.InnerHtml = str.ToUpper(); } </script> <!-- Layout --> <html> <head> <title> Change to Upper Case </title> </head> <body> <h3> Conversion to Upper Case </h3> <form runat="server"> <input runat="server" id="mytext" type="text" /> <input runat="server" id="button1" type="submit" value="Enter..." OnServerClick="convertoupper"/> <hr /> <h3> Results: </h3> <span runat="server" id="changed_text" /> </form> </body> </html> |
Copy this file to the web server's root directory. Generally it is c:\inetput\wwwroot. Open the file from the browser to run it and it should generate following result:
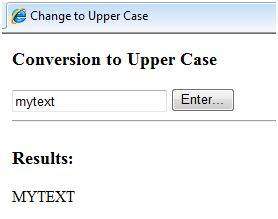
Using Visual Studio IDE:
Let us develop the same example using Visual Studio IDE. Instead of typing the code, you can just drag the controls into the design view:
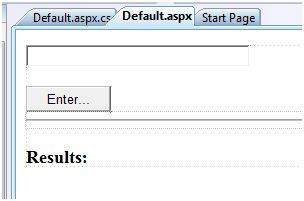
The content file is automatically developed. All you need to add is the Button1_Click routine, which is as follows:
protected void Button1_Click(object sender, EventArgs e) { string buf = TextBox1.Text; changed_text.InnerHtml = buf.ToUpper(); } |
The content file code is:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="firstexample._Default" %> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" > <head runat="server"> <title>Untitled Page</title> </head> <body> <form id="form1" runat="server"> <div> <asp:TextBox ID="TextBox1" runat="server" Width="224px"> </asp:TextBox> <br /> <br /> <asp:Button ID="Button1" runat="server" Text="Enter..." Width="85px" onclick="Button1_Click" /> <hr /> <h3> Results: </h3> <span runat="server" id="changed_text" /> </div> </form> </body> </html> |
Run the example either from Debug menu, or by pressing Ctrl-F5 or by right clicking on the design view and choosing 'View in Browser' from the popup menu. This should generate following result:

This post is so interactive and informative.keep updating more information...
ReplyDeleteDot Net Training In Mumbai
Dot Net Training In Ahmedabad
.Net Coaching Centre In Kochi
Dot Net Training In Trivandrum
.Net Coaching In Kolkata
Great blog!!! The information was more useful for us... Thanks for sharing with us...
ReplyDeletewhy python
why is python so popular
Great Post. Very informative. Keep Sharing!!
ReplyDeleteApply Now for DotNet Training Classes in Noida
For more details about the course fee, duration, classes, certification, and placement call our expert at 70-70-90-50-90
This is an awesome post. Really very informative and creative contents. Visit my website to get best Information About Best IAS coaching Institutes in Dadar.
ReplyDeleteBest IAS coaching Institutes in Dadar
Top IAS coaching Institutes in Dadar
This blog is so cool. I am learning dot net course online. This blogs helps me find out better understanding about salesforce training. Thank you for this blog!
ReplyDeleteLakshya IAS Academy is a well-known Institute and the Best IAS Coaching in Mumbai preparing candidates for the Civil Services Examination. It is one of the known IAS coaching centers in Mumbai, which is known for offering high-quality guidance to aspirants.
ReplyDeleteThanks for sharing this informative article on What is ASP.Net? If you want to ASP.Net Development Company for your project. Please visit us.
ReplyDelete